Creating a custom java trigger API for Infor Process Automation
This topic describes to create a custom API to be used to trigger a process.
The main classes of the API are:
-
LPSSession
-
ProcessRequest
-
ProcessResponse
The subsections of this topic describe each and provide examples.
System prerequisites
To build and run a Java program that uses the API, the following Lawson jar files must be added to the classpath.
In addition, if you plan to run these APIs on any machine other than the Lawson server, copy these files to that machine.
LAENVDIR/java/thirdParty/sec-client.jar
LAENVDIR/java/jar/bpm-interfaces.jar
LAENVDIR/java/jar/type.jar
LAENVDIR/java/jar/security.jar
LAENVDIR/java/jar/clientsecurity.jar
LAENVDIR/java/thirdParty/grid-client.jar
LAENVDIR/java/Lawutil_logging.jar
Starting and ending a session (LPSSession)
The LPSSession object keeps track of the communication session with the Infor Process Automation Server. A session object is created using the factory method createGridSession, passing the grid host, grid port, SSO user, SSO password, and Infor Process Automation data area. This call starts the session:
LPSSession session = LPSSession.createGridSession(
gridHost, gridPort, user, password, pfiDataArea);
When you are finished making calls to Infor Process Automation, use this call to end the session.
session.close();
Making requests
To make a request, first a ProcessRequest object is created:
ProcessRequest request = new ProcessRequest();
The next call sets properties. You can set the service name or the process name. If you set the service name, the process(s) run will be determined based on the service setup.
If you specify the flow name, the flow specified will be run:
request.setFlowName("myFlow");
When the request object is set up, a method of the LPSSession object is used to send the request to Infor Process Automation:
ProcessResponse response = session.runRequest(request, true);
The program should look at the results from running the flow, which are contained in the returned ProcessFlowResponse object. The runRequest method is used to make synchronous requests.
The main method for making an asynchronous request is:
createAndReleaseWorkunit: ProcessFlowResponse response = session.createAndReleaseWorkunit(request);
Simple example
Following is an example of simple Java program to run a process synchronously.
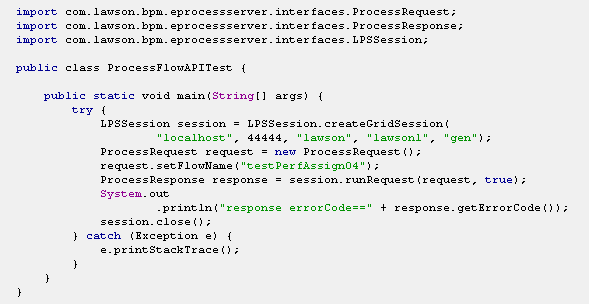