prerowupdate
Use prerowupdate
and postrowupdate
with objProperty
and objPropertySet
. You can make changes as a result of a
row being changed.
This prerowupdate
script tests the value of the QUANTITY field. Then, it uses
objPropertySet
to update the SECTION column for the row that was changed. Both
changes to the row are saved when the object is saved. The ingr
detail code precedes the prerowupdate
function.
Option Strict Off
imports System
imports System.Data
imports System.Diagnostics
Imports Microsoft.VisualBasic
Class HookScript
Inherits FcProcFuncSetEventHook
Function ingrprerowupdate() As Long
Dim qty As Double = FcType.FixDouble(objProperty("Quantity.Ingr"))
If qty > 100 Then
objPropertySet("BIG", 0, "SECTIONNAME.INGR")
Else
objPropertySet( "Small", 0, "SECTIONNAME.INGR")
End If
Return 1
End Function
end Class
In this example, the quantity in row 6 changed. Consequently, a change is made to the Section column before the object is saved.
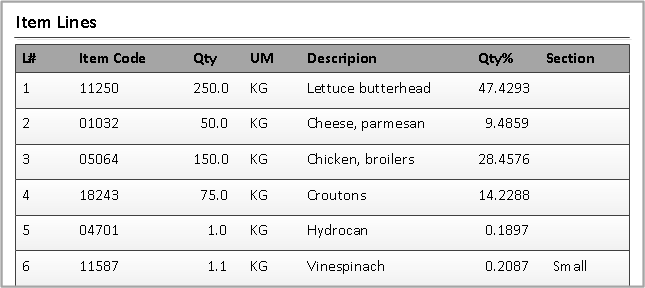
The postrowupdate
function updates related
information outside the row that depends on the row update being successful. You cannot
change the value in a row using postrowupdate
.
DataRowCurrent
and DataRowProposed
are not available in
the secured scripting environment. They have been replaced by
GetCurrentRowValue
, GetProposedRowValue
, and
SetProposedRowValue
.
You can use GetCurrentRowValue
, GetProposedRowValue
, and SetProposedRowValue
to access a single value on the row, not the entire row.
This is quicker than using ObjProperty
and ObjPropertySet
for this type of work.
Option Strict Off
Imports System
Imports System.Data
Imports System.Diagnostics
Imports Microsoft.VisualBasic
Class HookScript
Inherits FcProcFuncSetEventHook
Function ingrprerowupdate() As Long
Dim currVal as Object = Context.GetCurrentRowValue("UOM_CODE")
MessageList("currVal = " & currVal.ToString())
Dim propVal as Object = Context.GetProposedRowValue("UOM_CODE")
MessageList("propVal = " & propVal.ToString())
Context.SetProposedRowValue("UOM_CODE", "LB") ' This change will be honored
End Function
End Class
Additionally, you can return a negative value from your
PreRowUpdate
script hook. The RowUpdate
process is
halted by the system.
Function ingrprerowupdate() As Long
…
MessageList("Error processing Ingredient Row Update script.")
Return -1
End Function