Returning single values
These examples are for a single value being returned.
Retrieving the protein parameter
In this example, the VALIDATION_SUBCODE
contains a value, PROTEIN
. Consequently, all
of the arguments are needed.
To get the value for the COLUMN KEY:
- Find the property you want (for example,
PROTEIN
). - Look for
KEYCODE
with a VALIDATION_CODE that matches the one for the property. - Divide by 100.
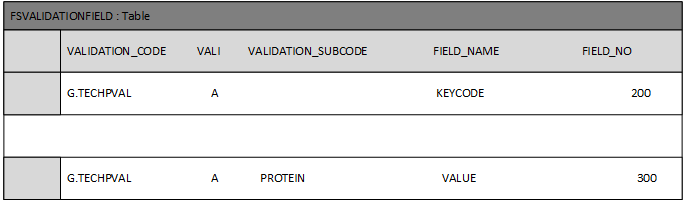
The function statement to retrieve the value of the Protein parameter is:
Dim oProtein1As Object = ObjProperty("VALUE.TPALL","","", "PROTEIN",2)
Dim dProtein2 As Double = cdbl(oProtein1)
Retrieving a status value
This example places the status (STATUSIND.STATUS
) of
the formula
PIZZA_SAUCE\003
into the local variable
oStatus
.
Dim oStatus As Object = objProperty("STATUSIND.STATUS",
"FORMULA","PIZZA_SAUCE\003")
When the status is too low, the user is notified in the alert box.
if (oStatus < 400) then
MessageList("This formula not approved. Status: ", oStatus)
end if
Retrieving the owner and the group
The owner and the group codes are retrieved for the current item
(OWNERCODE.PER, GROUPCODE.PER
). Those codes are placed into
the local variable
oOwner, oGroup
.
Dim oOwner As Object = ObjProperty("OWNERCODE.PER", "", "", _objectkey,
"ITEM_CODE")
Dim oGroup As Object = ObjProperty("GROUPCODE.PER", "", "", _objectkey,
"ITEM_CODE")
Return 111
Testing for a blank status value
You can do a comparison on a retrieved value. The retrieved value can be
blank if a user removed the value or did not enter the value. In this case,
add IsBlank
to your script. IsBlank
specifically tests for blank values.
In this example, the value of the VENDORSTATUS extension field is placed
into the local variable oVStat.
Dim oVStat As Object
Dim sVStatStr As String
Dim lMessage As Long
Because this is an extension field, the detail syntax is not needed.
Dim oVStat As Object = ObjProperty("VENDORSTATUS","FORMULA", "PIZZA\003")
Check if the field is blank or not approved.
if (IsBlank(oVStat ) = 1) Or (oVStat <> "APPROVED" ) then
MessageList("Vendor Status is not entered.")
Return 9111
end if
Retrieving an item status to specify the formula status
This example retrieves the lowest status value of all items in the formula and compares it to the status of the formula. If the status of the formula is greater than the lowest item status, then the formula status is set to the lowest item status value.
00
. See the Infor PLM for Process
Application Configuration Guide.
Dim oIngrStatus As Object = ObjMethod("","", "INGRLOWESTSTATUS")
Dim oFStatus As Object = ObjProperty("STATUSIND.STATUS","","")
if (oIngrStatus < oFStatus then
MessageList("Formula status is lower than item’s status.")
MessageList("Formula status set to lowest ingred status.")
ObjPropertySet(oingrStatus,0,"STATUSIND.STATUS")
end if
If Strict is On, replace the “if” statement that is shown above with this code:
if (CDbl(oIngrStatus) < CDbl(oFStatus)) then